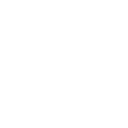
LineSeries - Interpolated Points
This discussion was imported from CodePlex
Artiga wrote at 2013-09-04 16:04:
Hello guys!
For my application I need the all points of a Line Series ... let me explain better:
Suppose I give two points to my Line Series, (0,0) and (10,10), OxyPlot will automatically plot the points between this two points.
It´s possible that I get this point list (the interpolated ones) ? For me will be nice, otherwise I will has to add a interpolate algorithm just to calculate then ....
Thx all
For my application I need the all points of a Line Series ... let me explain better:
Suppose I give two points to my Line Series, (0,0) and (10,10), OxyPlot will automatically plot the points between this two points.
It´s possible that I get this point list (the interpolated ones) ? For me will be nice, otherwise I will has to add a interpolate algorithm just to calculate then ....
Thx all
objo wrote at 2013-09-04 20:05:
The smoothed points is a private member of the LineSeries class. I don't want to expose this.
You find the algorithm for the canonical splines in CanonicalSplineHelper.cs
You find the algorithm for the canonical splines in CanonicalSplineHelper.cs
EvaldsUrtans wrote at 2013-11-01 16:24:
If you would like to add more points from key points in lineseries here's code
/// <summary>
/// Interpulate LineSeries data points for linear slopes
/// </summary>
public static List<IDataPoint> InterpulateLinearLines(List<IDataPoint> points, double intervalX)
{
List<IDataPoint> pointsReturn = new List<IDataPoint>();
double x = 0.0;
double xPrev = -1.0;
while (x != xPrev)
{
xPrev = x;
IDataPoint point1 = null;
IDataPoint point2 = null;
foreach (IDataPoint pointKey in points)
{
if (pointKey.X <= x)
{
point1 = pointKey;
}
else if (point1 != null)
{
point2 = pointKey;
break;
}
}
if (point2 == null)
{
point2 = points[points.Count - 1];
}
{
double sideA1 = point2.Y - point1.Y;
double sideA2 = 0.0f;
double sideB1 = point2.X - point1.X;
double sideB2 = x - point1.X;
if (sideB1 > 0.0)
{
IDataPoint pointCalc = new DataPoint();
sideA2 = (sideA1 * sideB2) / sideB1;
pointCalc.Y = point1.Y + sideA2;
pointCalc.X = x;
if(pointsReturn.Count > 0)
{
foreach (IDataPoint pointKey in points)
{
if(pointsReturn[pointsReturn.Count -1].X < pointKey.X)
{
if (pointKey.X < pointCalc.X)
{
pointsReturn.Add(pointKey);
}
break;
}
}
}
pointsReturn.Add(pointCalc);
}
else
{
pointsReturn.Add(point1);
}
}
x += intervalX;
x = Math.Min(x, points[points.Count - 1].X);
}
return pointsReturn;
}
usage:lineSeries.Points = HelperGraphs.InterpulateLinearLines(dataPointsRough, fTimeInterval);
Customer support service by UserEcho