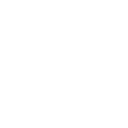
Mouse interact doesn't work with HitTest
This discussion was imported from CodePlex
sharethl wrote at 2012-07-13 14:41:
I created a new class inherit from Rectangle, and override HitTest in order to know user click which point of a rectangle, however, if I override HitTest, the mouse event can not fire at all.
Once the HitTest is deleted, Mouse event can be fired.
How to solve this problem?
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace OxyPlot.Extended.Annotations { public class RectangleAnnotationMovable2:RectangleAnnotation { private DataPoint lastPoint = DataPoint.Undefined; private OxyRect screenRectangle; private int handleNumber; private OxyColor originalStroke; private double originalStrokeThickness; private OxyColor originalFill; protected override void OnMouseDown(object sender, OxyMouseEventArgs e) { if ( this.PlotModel == null) return; handleNumber = (int)e.HitTestResult.Index; lastPoint = this.InverseTransform(e.Position); originalFill = this.Fill; this.Fill = OxyColors.Red; this.PlotModel.InvalidatePlot(false); e.Handled = true; } protected override void OnMouseMove(object sender, OxyMouseEventArgs e) { if (this.PlotModel == null) return; var thisPoint = this.InverseTransform(e.Position); double dx = thisPoint.X - lastPoint.X; double dy = thisPoint.Y - lastPoint.Y; switch (handleNumber) { case 1: this.MinimumX += dx; this.MaximumY += dy; break; case 2: this.MaximumY += dy; break; case 3: this.MaximumX += dx; this.MaximumY += dy; break; case 4: this.MaximumY += dy; break; case 5: this.MaximumX += dx; this.MinimumY += dy; break; case 6: this.MinimumY += dy; break; case 7: this.MinimumX += dx; this.MinimumY += dy; break; case 8: this.MinimumX += dx; break; } lastPoint = thisPoint; this.PlotModel.InvalidatePlot(false); e.Handled = true; } protected override void OnMouseUp(object sender, OxyMouseEventArgs e) { if (this.PlotModel == null) return; this.Fill = originalFill; } /// <summary> /// Tests if the plot element is hit by the specified point. /// </summary> /// <param name="point"> /// The point. /// </param> /// <param name="tolerance"> /// The tolerance. /// </param> /// <returns> /// A hit test result. /// </returns> protected override HitTestResult HitTest(ScreenPoint point, double tolerance) { if (screenRectangle.Contains(point)) { return new HitTestResult(point); } return null; } } }
objo wrote at 2012-08-09 00:39:
Thanks for reporting this, I added it as a bug http://oxyplot.codeplex.com/workitem/9977, will look into it later!
Customer support service by UserEcho