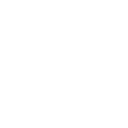
Rubber-banding with OxyPlot
This discussion was imported from CodePlex
mohitvashistha wrote at 2013-05-06 14:35:
I want to rubberband a line series as we rubberband a polyline in any CAD application.
I have written an example for the same.
Please suggest why mouse down is not fires while rubber banding.
Also how can i get the ESC key to end the rubberbanding.
I have written an example for the same.
[Example("LineSeries rubberbanding")]
public static PlotModel MouseRubberbandingEvent()
{
var model = new PlotModel("Rubberbanding",
"Left click to add line and press Esc to end.")
{
LegendSymbolLength = 40
};
// Add a line series
var s1 = new LineSeries("LineSeries1")
{
Color = OxyColors.SkyBlue,
MarkerType = MarkerType.Circle,
MarkerSize = 6,
MarkerStroke = OxyColors.White,
MarkerFill = OxyColors.SkyBlue,
MarkerStrokeThickness = 1.5
};
model.Series.Add(s1);
s1.Points.Add(new DataPoint(10,
10));
IDataPoint tempDataPoint = new DataPoint(0,0);
s1.Points.Add(tempDataPoint);
// Remember to refresh/invalidate of the plot
model.RefreshPlot(false);
bool isRubberbanding = false;
// Subscribe to the mouse down event on the line series
model.MouseDown += (s, e) =>
{
// only handle the left mouse button (right button can still be used to pan)
if (e.ChangedButton == OxyMouseButton.Left)
{
s1.Points.Add(s1.InverseTransform(e.Position));
isRubberbanding = true;
// Remember to refresh/invalidate of the plot
model.RefreshPlot(false);
// Set the event arguments to handled - no other handlers will be called.
e.Handled = true;
}
};
// Subscribe to the mouse down event on the line series
s1.MouseDown += (s, e) =>
{
// only handle the left mouse button (right button can still be used to pan)
if (
(e.ChangedButton == OxyMouseButton.Left)
&&
(isRubberbanding)
)
{
s1.Points.Add(s1.InverseTransform(e.Position));
isRubberbanding = true;
// Remember to refresh/invalidate of the plot
model.RefreshPlot(false);
// Set the event arguments to handled - no other handlers will be called.
e.Handled = true;
}
};
model.MouseMove += (s, e) =>
{
if (isRubberbanding)
{
var point = s1.InverseTransform(new ScreenPoint(e.Position.X-8,
e.Position.Y-8));
tempDataPoint.X = point.X;
tempDataPoint.Y = point.Y;
s1.Points.Remove(tempDataPoint);
s1.Points.Add(tempDataPoint);
model.RefreshPlot(false);
}
};
model.MouseUp += (s, e) =>
{
if (isRubberbanding)
{
s1.LineStyle = LineStyle.Solid;
model.RefreshPlot(false);
e.Handled = true;
}
};
return model;
}
It works fine when I offset the mouse cursor by 8 pixel. But when I don't do that the mouse down event doesn't fire up neither for plot model nor for line series.Please suggest why mouse down is not fires while rubber banding.
Also how can i get the ESC key to end the rubberbanding.
Customer support service by UserEcho