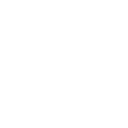
simple TextAnnotation
This discussion was imported from CodePlex
heromyth wrote at 2011-11-03 08:12:
I need some text annotations for my curves, so I implemented a simple TextAnnotation.
public partial class Form1 : Form { public Form1() { InitializeComponent(); var pm = new PlotModel("Trigonometric functions", "Example using the FunctionSeries") { PlotType = PlotType.Cartesian, Background = OxyColors.White }; pm.Series.Add(new FunctionSeries(Math.Sin, -10, 10, 0.1, "sin(x)")); pm.Series.Add(new FunctionSeries(Math.Cos, -10, 10, 0.1, "cos(x)")); pm.Series.Add(new FunctionSeries(t => 5 * Math.Cos(t), t => 5 * Math.Sin(t), 0, 2 * Math.PI, 0.1, "cos(t),sin(t)")); TextAnnotation ta = new TextAnnotation(); DataPoint dp = new DataPoint(); dp.X = 1; dp.Y = 3.5; ta.addPoint(dp); dp = new DataPoint(); dp.X = 21; dp.Y = 4.5; ta.addPoint(dp); pm.Annotations.Add(ta); plot1.Model = pm; } }public class TextAnnotation : Annotation { private IList<IDataPoint> dataPoints; public void addPoint(IDataPoint dp) { dataPoints.Add(dp); } /// <summary> /// Vertical alignment of text (above or below the line). /// </summary> public VerticalTextAlign TextVerticalAlignment { get; set; } /// <summary> /// Horizontal alignment of text. /// </summary> public HorizontalTextAlign TextHorizontalAlignment { get; set; } public override void Render(IRenderContext rc, PlotModel model) { double angle = 0; ScreenPoint position = new ScreenPoint(); foreach (IDataPoint dp in dataPoints) { if (dp.X > this.XAxis.ActualMaximum || dp.X < this.XAxis.ActualMinimum) continue; if (dp.Y > this.YAxis.ActualMaximum || dp.Y < this.YAxis.ActualMinimum) continue; position.X = this.XAxis.Transform(dp.X); position.Y = this.YAxis.Transform(dp.Y); string text = dp.Y.ToString("F1"); rc.DrawText( position, text, model.TextColor, model.ActualAnnotationFont, model.AnnotationFontSize, FontWeights.Normal, angle, this.TextHorizontalAlignment, this.TextVerticalAlignment); } } public TextAnnotation() { this.TextHorizontalAlignment = HorizontalTextAlign.Center; this.TextVerticalAlignment = VerticalTextAlign.Top; dataPoints = new List<IDataPoint>(50); } }
objo wrote at 2011-11-03 22:53:
cool, I will add a TextAnnotation class, but I think we should make it a bit more general (not only showing Y value).
I think the input properties should be a single point, horizontal/vertical alignment and a text string.
Служба підтримки клієнтів працює на UserEcho