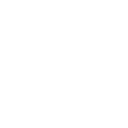
ColumnSeries doesn't show the data
This discussion was imported from CodePlex
rchan510 wrote at 2014-03-12 23:18:
Can someone please advise what's wrong with my code? it is a very simple test to create a column chart but whatever I do I can only see the chart without any columns (no data).
using OxyPlot;using OxyPlot.Axes;
using OxyPlot.Series;
using System;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Diagnostics;
namespace test
{
public class MainViewModel : ViewModelBase
{
private ObservableCollection<ReportModel> content;
public ObservableCollection<ReportModel> Content { get { return content; } set { content = value; OnPropertyChanged("Content"); } }
private PlotModel plotModel;
public PlotModel PlotModel { get { return plotModel; } set { plotModel = value; OnPropertyChanged("PlotModel"); } }
public MainViewModel()
{
Content = new ObservableCollection<ReportModel>();
PlotModel = new PlotModel();
SetupContent();
SetupModel();
}
private void SetupModel()
{
var dateAxis = new DateTimeAxis(AxisPosition.Bottom, "Date", "MM/yy")
{
MajorGridlineStyle = LineStyle.Solid,
MinorGridlineStyle = LineStyle.Dot,
IntervalLength = 80
};
//PlotModel.Axes.Add(dateAxis);
var valueAxis = new LinearAxis(AxisPosition.Left, 0)
{
MajorGridlineStyle = LineStyle.Solid,
MinorGridlineStyle = LineStyle.Dot,
Minimum = 13000,
Maximum = 16000,
Title = "Value"
};
//plotModel.Axes.Add(valueAxis);
var lineSeries = new ColumnSeries() { ItemsSource = Content, ValueField = "Amount" };
PlotModel.Series.Add(lineSeries);
}
private void SetupContent()
{
Content.Add(new ReportModel("12/1/2013", 14000));
Content.Add(new ReportModel("1/1/2014", 15000));
Content.Add(new ReportModel("2/1/2014", 14500));
}
}
public class ReportModel : ViewModelBase
{
private DateTime date;
public DateTime Date { get { return date; } set { date = value; OnPropertyChanged("Date"); } }
private int amount;
public int Amount { get { return amount; } set { amount = value; OnPropertyChanged("Amount"); } }
public ReportModel(string date, int amount)
{
Date = Convert.ToDateTime(date);
Amount = amount;
}
}
public abstract class ViewModelBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propName)
{
Debug.Assert(GetType().GetProperty(propName) != null);
var pc = PropertyChanged;
if (pc != null)
pc(this, new PropertyChangedEventArgs(propName));
}
}
}
objo wrote at 2014-03-13 07:08:
I think you must use a
CategoryAxis
and not a DateTimeAxis
. Do you get an exception? it should be thrown by the ColumnSeries.GetCategoryAxis.rchan510 wrote at 2014-03-13 22:24:
Here is the new code. Actually I have tried with or without CategoryAxis and LinearAxis. The result is similar and only show the empty chart...
using OxyPlot;
using OxyPlot.Axes;
using OxyPlot.Series;
using System;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Diagnostics;
namespace test
{
public class MainViewModel : ViewModelBase
{
private ObservableCollection<ReportModel> content;
public ObservableCollection<ReportModel> Content { get { return content; } set { content = value; OnPropertyChanged("Content"); } }
private PlotModel plotModel;
public PlotModel PlotModel { get { return plotModel; } set { plotModel = value; OnPropertyChanged("PlotModel"); } }
public MainViewModel()
{
Content = new ObservableCollection<ReportModel>();
PlotModel = new PlotModel();
SetupContent();
SetupModel();
}
private void SetupModel()
{
var dateAxis = new CategoryAxis() { ItemsSource = Content, LabelField = "Date" };
PlotModel.Axes.Add(dateAxis);
var valueAxis = new LinearAxis(AxisPosition.Left, 0);
PlotModel.Axes.Add(valueAxis);
var lineSeries = new ColumnSeries() { ItemsSource = Content, ValueField = "Amount" };
PlotModel.Series.Add(lineSeries);
}
private void SetupContent()
{
Content.Add(new ReportModel("12/1/2013", 14000));
Content.Add(new ReportModel("1/1/2014", 15000));
Content.Add(new ReportModel("2/1/2014", 14500));
}
}
public class ReportModel : ViewModelBase
{
private DateTime date;
public DateTime Date { get { return date; } set { date = value; OnPropertyChanged("Date"); } }
private int amount;
public int Amount { get { return amount; } set { amount = value; OnPropertyChanged("Amount"); } }
public ReportModel(string date, int amount)
{
Date = Convert.ToDateTime(date);
Amount = amount;
}
}
public abstract class ViewModelBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propName)
{
Debug.Assert(GetType().GetProperty(propName) != null);
var pc = PropertyChanged;
if (pc != null)
pc(this, new PropertyChangedEventArgs(propName));
}
}
}
rchan510 wrote at 2014-03-16 22:40:
I am having issue on generating a Column/Bar Chart since upgraded to VS2013. After spending days on searching solution from web and testing, I finally believe it doesn't work in the new OxyPlot package...
Below is the test code. It works when I use the NoPCL.2013.1.51.1 version, however when I replaced the OxyPlot by downloading the latest version from NuGet (version 2014.1.249.1) it shows only an empty chart (only show the axes but no data or no column).
Below is the test code. It works when I use the NoPCL.2013.1.51.1 version, however when I replaced the OxyPlot by downloading the latest version from NuGet (version 2014.1.249.1) it shows only an empty chart (only show the axes but no data or no column).
- View
<Window x:Class="test.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:oxy="http://oxyplot.codeplex.com"
Title="MainWindow" Height="350" Width="525">
<Grid>
<oxy:Plot Model="{Binding PivotData}" />
</Grid>
</Window>
- ViewModel
using OxyPlot;
using OxyPlot.Axes;
using OxyPlot.Series;
using System;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Globalization;
namespace test
{
public class MainViewModel : ViewModelBase
{
private ObservableCollection<SalesModel> salesData;
public ObservableCollection<SalesModel> SalesData
{
get { return salesData; }
set { salesData = value; OnPropertyChanged("SalesData"); }
}
private PlotModel pivotData;
public PlotModel PivotData
{
get { return pivotData; }
set { pivotData = value; OnPropertyChanged("PivotData"); }
}
public MainViewModel()
{
GenerateSalesData();
CreatePivotChart();
UpdatePivotData();
}
private void GenerateSalesData()
{
SalesData = new ObservableCollection<SalesModel>();
SalesData.Add(new SalesModel(new DateTime(2013, 11, 1), 12000));
SalesData.Add(new SalesModel(new DateTime(2013, 12, 1), 13000));
SalesData.Add(new SalesModel(new DateTime(2014, 1, 1), 12500));
SalesData.Add(new SalesModel(new DateTime(2014, 2, 1), 14000));
}
private void CreatePivotChart()
{
PivotData = new PlotModel();
PivotData.Title = "Sales Monthly Summary";
}
private void UpdatePivotData()
{
var axisX = new CategoryAxis(AxisPosition.Bottom) { ItemsSource = SalesData, LabelField = "Date" };
PivotData.Axes.Add(axisX);
var axisY = new LinearAxis(AxisPosition.Left) { Title = "USD" };
PivotData.Axes.Add(axisY);
var dataSeries = new ColumnSeries() { ItemsSource = SalesData, ValueField = "Amount" };
PivotData.Series.Add(dataSeries);
}
}
public class SalesModel : ViewModelBase
{
private string date;
public string Date { get { return date; } set { date = value; OnPropertyChanged("Date"); } }
private int amount;
public int Amount { get { return amount; } set { amount = value; OnPropertyChanged("Amount"); } }
public SalesModel(DateTime _date, int _amount)
{
date = new DateTimeFormatInfo().GetAbbreviatedMonthName(_date.Month) + "/" + (_date.Year).ToString();
amount = _amount;
}
}
public abstract class ViewModelBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void OnPropertyChanged(string prop)
{
var pc = PropertyChanged;
if (pc != null)
pc(this, new PropertyChangedEventArgs(prop));
}
}
}
objo wrote at 2014-03-19 08:32:
Thanks for providing the code, does anyone see the problem?
Customer support service by UserEcho