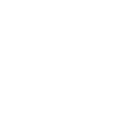
Linking data series and annotations
my lets say length of my int[] data is 15 and it contains values {22, 44, 55, 87, 33, 21, 23, 44, 33, 42, 54, 56, 66, 77, 99}
I need to place letter "H" over position 3, "Z" over position 8, and "T" over position 12. All annotations are near the top of the plot area. My code works fine displaying regular LineSeries but I cant figure out how to add the annotations. Any help is appreciated
public void SetWaveformData(int[] data)
{
PlotModel plotModel = new PlotModel();
List<DataPoint> dataSeries = new List<DataPoint>();
int i = 0;
foreach (int yValue in data)
{
dataSeries.Add(new DataPoint { X = i++, Y = yValue });
}
LineSeries ser = new LineSeries();
ser.Points.AddRange(dataSeries);
plotModel.Series.Add(ser);
}
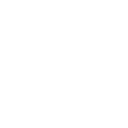
Set ItemsSource to a Collection of IDataPoints.
willmoore88 wrote at 2013-10-21 17:42:
public Collection<IDataPoint> dataPoints1 = new Collection<IDataPoint>();
I set my ScatterSeries too said Collection.var lineSeries1 = new ScatterSeries((string)Application.Current.FindResource("cloudLayersText"))
{
MarkerFill = CloudDetColour,
MarkerStroke = CloudDetColour,
MarkerType = MarkerType.Square,
MarkerSize = 1.5,
ItemsSource = dataPoints1,
};
Why is my graph not showing the data I then subsequently add to my collection of IDataPoints? I know there is data in there as I can see there is over 1400 items in the collection.willmoore88 wrote at 2013-10-23 11:18:
objo wrote at 2013-10-23 11:23:
willmoore88 wrote at 2013-10-23 11:26:
CloudLayerPlotModel.RefreshPlot(true);
OnPropertyChanged("CloudLayerPlotModel");
willmoore88 wrote at 2013-10-24 09:45:
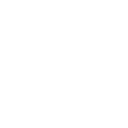
Writing PDF report to a FileStream
ambrein2 wrote at 2014-01-16 04:10:
I am new to OxyPlot and still getting to know OxyPlot more. I recently was able to create a Plot that contains LineSeries and now I am trying to create a PDF report of this plot. However I don't want to write this report to an actual file, in fact I want to write the PDF report directly to a FileStream. Is there an easier way to do this with OxyPlot library?
objo wrote at 2014-01-16 22:57:
https://oxyplot.codeplex.com/workitem/10116
ambrein2 wrote at 2014-01-21 01:54:
objo wrote at 2014-02-02 20:50:
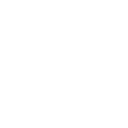
Plot not filling parent container (WPF)
nsudac wrote at 2013-05-24 11:12:
I have an OxyPlot inside a WPF Viewbox, something like the following:
<Viewbox Name="ViewBig">
<oxy:Plot Model="{Binding CameraGraph}" Name="OxyPlot" />
</Viewbox>
I would like the plot to automatically size to fit the Viewbox, as happens if I put an Image for example in the Viewbox. The problem I am having is that unless I explicitly set the Width and Height of the plot, it remains invisible. I know that the Binding to the model is working correctly as when I do set to the Width and Height explicitly the data is all there.
Am I missing something here?
Thanks in advance for any guidance.
objo wrote at 2013-06-08 12:13:
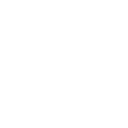
Vertical Annotations with DateTimeAxis
luisfrossi wrote at 2013-06-08 00:47:
I would like to add a few like annotations on a Plot with DateTimeAxis... the X value input at an LineAnnotation is a double value. How should i format my DateTime value to the X one?
Regards,
LR
luisfrossi wrote at 2013-06-08 01:03:
Just have to use OxyPlot Double conversion.
Thank you!
Great Work here.
objo wrote at 2013-06-08 10:51:
DateTimeAxis.ToDouble
or DateTimeAxis.CreateDataPoint
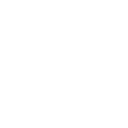
PdfSharp/OxyPlot.Pdf version mismatch
thomasdr wrote at 2013-10-22 18:50:
Could not load file or assembly 'PdfSharp, Version=1.31.1789.0, Culture=neutral, PublicKeyToken=f94615aa0424f9eb' or one of its dependencies. The located assembly's manifest definition does not match the assembly reference.
The current PdfSharp version is 1.32.2608.0. The version being using in OxyPlot.Pdf is from 2009. How can I reconcile this? Do I have to build OxyPlot.Pdf from the source code?
objo wrote at 2013-10-22 19:39:
thomasdr wrote at 2013-10-22 21:51:
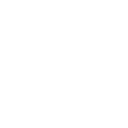
Logarithmic steps
willmoore88 wrote at 2013-08-07 12:11:
I have altered the logarithmic scale slightly to only draw major ticks and for them to be at intervals I choose.
https://www.dropbox.com/s/hge7bgbnz9ziz5t/back.png
objo wrote at 2013-08-07 12:47:
willmoore88 wrote at 2013-08-07 12:48:
willmoore88 wrote at 2013-08-07 12:49:
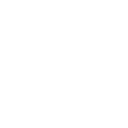
Update Graph After Zoom
kurtisharms wrote at 2013-01-29 07:54:
I am drawing functions using my own custom function generator by simply creating a new LineSeries of ~120 points and then plotting that between -10 and 10 to start with using Oxyplot's Windows 8 control. The use of my own custom function rather than a FunctionSeries is necessary because the function input has many variables and other oddities.
What I would like to do is the following:
1) Detect the end of a zooming event and bind an updateGraph() function to this. I cannot find this type of event. The mouse events don't appear to do the trick, as they are either continuous and generate too many events (ie. PointerWheelChanged) or don't work as I expected (ie. ManipulationCompleted).
2) Having detected the zooming event, updateGraph() updates the graph by creating a new LineSeries from my function that spans the new min to max displayed on the graph. I haven't successfully been able to find the new min/max displayed on the graph.
Any help would be greatly appreciated and thank you in advance!
objo wrote at 2013-02-07 22:37:
The ActualMaximum and ActualMinimum properties of the axes should also be set at this point.
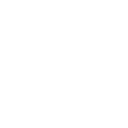
Multiple Panes Axis chart
meenakshi07 wrote at 2012-10-15 11:24:
Hi!
I like OxyPlot a lot and I love exploring it. thank you very much for your effort!
I am using Multiple Panes Axis chart in my project.
I have few queries on the same
1. If tracker lines color can be changed ? if yes, how ?
2. whenever I select a point on a lineseries, it shows vertical and Horizontal tracker lines. Is is possible to get trackerformatstring(in tooltip) for other lineseries where the tracker lines intersect them ? if yes, please let me know how we can do it ?
I apologise, if I have asked very basic question, as I am new to WPF itself
Thanks in Advance
Regards,
Meenakshi
objo wrote at 2012-10-16 23:38:
1. It should be possible to set the "DefaultTrackerTemplate" property of the Plot control. The default value is
<ControlTemplate> <local:TrackerControl Position="{Binding Position}" LineExtents="{Binding PlotModel.PlotArea}"> <local:TrackerControl.Content> <TextBlock Text="{Binding}" Margin="7" /> </local:TrackerControl.Content> </local:TrackerControl> </ControlTemplate>
See Source\Examples\WPF\WpfExamples\Examples\CustomTrackerDemo
The TrackerControl has properties for "LineStroke", "HorizontalLineVisibility" and "VerticalLineVisibility".
2. It is only tracking one series (finds the nearest when you click down, then follows this series), if you want values from other series you must calculate these yourself (oxyplot has few restrictions on the series and axes => it could be any number of intersections...)
meenakshi07 wrote at 2012-10-17 09:08:
Thanks for your help :)
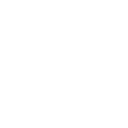
Multiple X-Axis with Shared Y-Axis
maddog56 wrote at 2014-03-02 02:48:

muzab wrote at 2014-03-04 09:49:
I can get a plot on only one x-y1 axis combination but not on the other x-y2,y3,y4.
muzab wrote at 2014-03-04 13:32:
maddog56 wrote at 2014-03-04 18:08:
Служба підтримки клієнтів працює на UserEcho