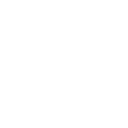
Possible bug: cannot zoom in on a Cartesian plot with multiple axes
[Example("Four axes")]
public static PlotModel FourAxes()
{
var plotModel1 = new PlotModel();
plotModel1.PlotType = PlotType.Cartesian;
plotModel1.Axes.Add(new LinearAxis { Maximum = 36, Minimum = 0, Title = "km/h" });
plotModel1.Axes.Add(new LinearAxis { Maximum = 10, Minimum = 0, Position = AxisPosition.Right, Title = "m/s" });
plotModel1.Axes.Add(new LinearAxis
{
Maximum = 10,
Minimum = 0,
Position = AxisPosition.Bottom,
Title = "meter"
});
plotModel1.Axes.Add(new LinearAxis
{
Maximum = 10000,
Minimum = 0,
Position = AxisPosition.Top,
Title = "millimeter"
});
return plotModel1;
}

Real time sample
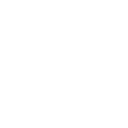
Movable (drag-able) Annotation
ChevyCP wrote at 2012-03-19 20:25:
Hi,
I have a wpf plot with multiple, user-selectable line series. The X axis is date time. I have two vertical annotation lines. I was wondering if it would be possible somehow to make those annotation lines drag-able along the X axis. I want to do this so the user can then re-run my evaluation software using data between those two date points.
Thanks!
ChevyCP wrote at 2012-03-19 21:37:
Basically these annotation lines would function as a slider, (time slider in my case).
objo wrote at 2012-03-20 18:35:
I would like to add MouseDown, MouseMove and MouseUp events on the PlotElement class - then it should be possible to handle mouse events on both annotations, axes and series. This should not be difficult, but it is a little bit of work to implement the hit testing (I would not depend on the presentation layer to do this (wpf/silverlight/winforms)).
ChevyCP wrote at 2012-03-22 21:00:
Isn't there hit testing already on the series, since there is tracker functionality?
Would it be easier to put hit testing/mouse events on the annotation class or create a custom annotation class that is based on a (re-skinned) slider control? (That way the needed functionality already exists.) Just a thought.
objo wrote at 2012-03-30 13:29:
Series: yes, I would use the code from the hit testing - probably some refactoring is needed.
I would like to add mouse events to the PlotElement class, and implement the hit testing on each of the derived classes (annotations, axes, series). The hit testing should be done in the OxyPlot library to make it platform independent (which means I would not use the hit testing of the WPF/Silverlight elements...)
I think the mouse events should be sufficient to make the annotation lines draggable, no need for Slider controls here.
sharethl wrote at 2014-03-06 03:22:
How to implement multiple annotations in one plot, and move one individually?
I try to for loop through all annotations and hittest each one, but hittest is protected.
Following code try to follow oxyplot design pattern, but have problem on finding which annotation currently focused.
Class PlotExample:Plot{ var arrow = new ArrowAnnotation(); arrow.StartPoint = new DataPoint(50, 0); arrow.EndPoint = new DataPoint(50, 100); arrow.MouseDown += NormalTool.OnMouseDown; arrow.MouseMove += NormalTool.OnMouseMove; arrow.MouseUp += NormalTool.OnMouseUp; this.Annotations.Add(arrow); } static class NormalTool { public static void OnMouseDown(object sender, OxyMouseEventArgs e) { if (e.ChangedButton == OxyMouseButton.Left) { // cannot access to arrow.... } } public static void OnMouseMove(object sender, OxyMouseEventArgs e) { } public static void OnMouseUp(object sender, OxyMouseEventArgs e) { } }
sharethl wrote at 2014-03-07 15:35:
made an new annotation that inherits from arrow annotation, and override mouse even in "new annotation" class.
In this way, don't need to use external tool class to modify annotation's properties.
public class SelectableArrowAnnotation:ArrowAnnotation { // any better name? DataPoint lastPoint = DataPoint.Undefined; bool moveStartPoint = false; bool moveEndPoint = false; OxyColor originalColor = OxyColors.White; protected override void OnMouseDown(object sender, OxyPlot.OxyMouseEventArgs e) { if (e.ChangedButton != OxyMouseButton.Left) { return; } lastPoint = this.InverseTransform(e.Position); moveStartPoint = e.HitTestResult.Index != 2; moveEndPoint = e.HitTestResult.Index != 1; originalColor = this.Color; this.Color = OxyColors.Red; var model = this.PlotModel; model.InvalidatePlot(false); e.Handled = true; } protected override void OnMouseMove(object sender, OxyPlot.OxyMouseEventArgs e) { var thisPoint = this.InverseTransform(e.Position); double dx = thisPoint.X - lastPoint.X; double dy = thisPoint.Y - lastPoint.Y; if (moveStartPoint) { this.StartPoint = new DataPoint(this.StartPoint.X + dx, this.StartPoint.Y + dy); } if (moveEndPoint) { this.EndPoint = new DataPoint(this.EndPoint.X + dx, this.EndPoint.Y + dy); } lastPoint = thisPoint; var model = this.PlotModel; model.InvalidatePlot(false); e.Handled = true; } protected override void OnMouseUp(object sender, OxyPlot.OxyMouseEventArgs e) { this.Color = originalColor; } }
wolf9s wrote at 2014-06-09 16:24:
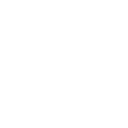
Random Color Picker
jpnavarini wrote at 2012-03-19 17:44:
I would like to create a trend with multiple LineSeries, however it would be necessary to pick a different color for each added LineSeries. Is there a color picker function to choose "random" colors, or I would have to do it manually?
objo wrote at 2012-03-20 18:26:
See the DefaultColor property of the PlotModel, you can change this to a list of random colors. The default value uses a predefined palette, not random colors.
ps. I will change the DefaultColor property to an IList<Color>
jpnavarini wrote at 2012-03-20 18:50:
When I add a new LineSeries to the Series list, it plots using the same default color (dark green). However, when I add multiple plots at once (inside the same loop, for example), each lineseries get a different color. That color rotation is what I wanted, however with lineseries added at different moments.
objo wrote at 2012-03-30 13:20:
Sorry, I don't quite understand. Is this a bug in the library? Do you add all series to the same plot?
I would like the plot always to generate the same colors (no randomness), if you want random colors to be generated when adding new series, this should be handled by your code.
JeanBisson wrote at 2014-01-09 17:27:
If you add a new LineSeries without its Color property set, the LineSeries will be assigned a Color (assigned to the ActualColor property) based on the number of LineSeries in the plot with its Color property still set to its default value (0x000001). Therefore, if you have 5 LineSeries in a plot of which 2 still have their Color property set to default, the Color assigned to the third LineSeries will be the third Color in the DefaultColors IList<> collection.
If you elect to assign a Color to a LineSeries (thereby assigning a non-default value to its Color property) and Refresh the plot, all other LineSeries may have their ActualColor property re-assigned a new color based on the number of LineSeries with default Colors remaining in the Series collection.
For example: First four colors in the DefaultColors IList<> collection are Red, Blue, Brown and Violet. The first LineSeries added with a default Color will have Red assigned to its ActualColor. Blue for the second, Brown for the third. Now, if you assign say Yellow to the second LineSeries and refresh the plot, you will find that the first LineSeries remains Red but the third LineSeries (previously Brown) will be re-assigned to Blue (since its the next in line in the IList<>).
In short, default / random colors are only assigned if their Color property is set to its default value of 0x000001. Once added to a plot, updating its Color can affect the color of other LineSeries (defpending on their order in the Series collection with regard to other LineSeries with a default Color property.
Hope this helps and thank you for this excellent library.
Cheers,
Jean
objo wrote at 2014-01-10 09:20:
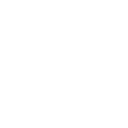
Panning with Category Axis
bedidos wrote at 2012-05-26 02:28:
This library is great!
Is it possible to pan with a category axis? So far, I have not been able to get panning to work on a category axis using latest source code.
objo wrote at 2012-05-26 08:24:
Yes, it is now! I fixed the bug (a simple change in CategoryAxis.UpdateActualMaxMin)
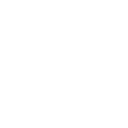
Possible bug: zoom and smooth
everytimer wrote at 2013-09-04 23:14:
Is there something that can be done to solve this?
PD. I've tested and even if you don't zoom at the Smoothed LineSeries but to an empty place you'll get the same problem.
objo wrote at 2013-09-05 07:53:
Yes, I think the line should be clipped before smoothing. But we need a new clipping algorithm, which should include some points outside the clipping rectangle, otherwise the spline will be interpolated differently.
I have added it to the issues:
https://oxyplot.codeplex.com/workitem/10075
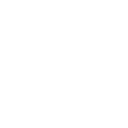
Can't display oxyplot in Xaml.
MartijnCarol wrote at 2013-10-30 16:32:
xmlns:oxy="clr-namespace:OxyPlot.Wpf;assembly=OxyPlot.Wpf"
and <oxy:Plot Margin="0" Grid.Column="1" Model="{Binding ViewModelTest.ChartModelECG, Source={StaticResource Locator}}"/> />
But keep getting this errorMethod 'DrawEllipse' in type 'OxyPlot.Wpf.ShapesRenderContext' from assembly 'OxyPlot.Wpf, Version=2013.2.107.1, Culture=neutral, PublicKeyToken=75e952ba404cdbb0' does not have an implementation
If I change the nugetpacked to OxyPlot.Wpf_noPCL can see the chart in Xaml but cant start the prodject due to conflicts with he mvvm-light libery where as soon as I add plotModel1 = new PlotModel(); the following error acures
An exception of type 'System.Reflection.TargetInvocationException' occurred in mscorlib.dll but was not handled in user code
anny Idea what i'm dooing wrong?
Martijn Carol
_Noctis_ wrote at 2013-11-01 10:07:
Did you try downloading the dll's, and just adding them to your references in the project ? (I think this is what I did ... )
_Noctis_ wrote at 2013-11-04 14:01:
Did you try installing the Nuget, cleaning the project, closing the VS and opening it again ?
(Seems it worked for some people, and between this and actually installing local dll's , it might have been the solution ...)
richardfen wrote at 2013-11-04 20:01:
http://oxyplot.codeplex.com/releases/view/76035
download the zip file and extract all the DLL's. I took the 4.5 versions and referenced the following:
OxyPlot.dll
OxyPlot.Wpf
OxyPlot.Xps.dll
The program that I used the above to build successfully was SimpleDemo. You can get this sample by doing to:
https://oxyplot.codeplex.com/SourceControl/latest#readme.txt
Then from the tree select: Source | Examples | WPF | SimpleDemo
objo wrote at 2013-11-05 19:00:
This should be fixed from version 2013.2.114.1!
akmm94 wrote at 2014-06-20 04:40:
When I am calling a new window to show up Oxy plot it just does not show up at all. If I open the window by itself it shows the plot. But if I call from another window it just does not show up. What can be the reason? Any help would be appreciated.
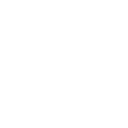
Xaml binding isnt working....
VadimTr wrote at 2013-10-21 22:49:
When i create graph in XAML, then binding to observable collection - it isn't working.
Why?
<oxy:Plot Margin="10">
<oxy:LineSeries x:Name="OxyPlotLine"
Width="Auto"
Height="Auto" ItemsSource="{Binding Mode=OneWay, Source={StaticResource ChartPoints}}" />
</oxy:Plot>
for (double i = 0; i <= stopValue; i++)
{
ii =+ i;
CPColl.Add(new ChartPoint(i, CPColl.Count););
}
class ChartPoint
{
private double _X;
private double _Y;
public ChartPoint(double X, double Y)
{
_X = X;
_Y = Y;
}
public double X
{ get { return _X; } set { _X = value; } }
public double Y
{ get { return _Y; } set { _Y = value; } }
}
class ChartPointsCollection: ObservableCollection<ChartPoint>
{
public ChartPointsCollection() : base()
{ }
}
objo wrote at 2013-10-22 22:55:
VadimTr wrote at 2013-10-22 23:14:
Can your write a sample?
VadimTr wrote at 2013-10-22 23:21:
objo wrote at 2013-10-23 07:59:
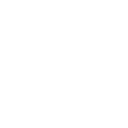
Making an axis invisible
dpru wrote at 2012-10-09 16:16:
How can I make an axis invisible? I have tried setting "IsAxisVisible" to false on each of my axes, but nothing happens when I do so (and yes, I am refreshing the plot). I am using WPF.
I have tried setting IsAxisVisible to false in both XAML and in code - I don't get anything happening with either way of doing things.
I simply want my axes to be black lines...no labels...nothing.
objo wrote at 2012-10-09 22:00:
I added an example using the IsAxisVisible, see "Axis examples | Invisible axis" examples in the example browsers. It is possible there is an error in the OxyPlot.Wpf.Axis class, but I couldn't reproduce the bug.
Service d'assistance aux clients par UserEcho